Session-Based Authentication vs JWT: Which Is Better for Your App?
When you’re building a web application, one of the key decisions is how to manage user authentication securely and efficiently. Two common approaches are Session-Based Authentication and JWT-Based Authentication. But how do they differ, and which one should you use for your app?
In this blog, we’ll break down these two approaches and discuss their pros and cons, giving you a better idea of which one might be the right fit for your project.
Session-Based Authentication
In Session-Based Authentication, user data is stored on the server in a session store, and the client is given a session ID. Think of it like getting a boarding pass with just a reference number—your flight details are stored elsewhere, and you only need the reference to retrieve them.
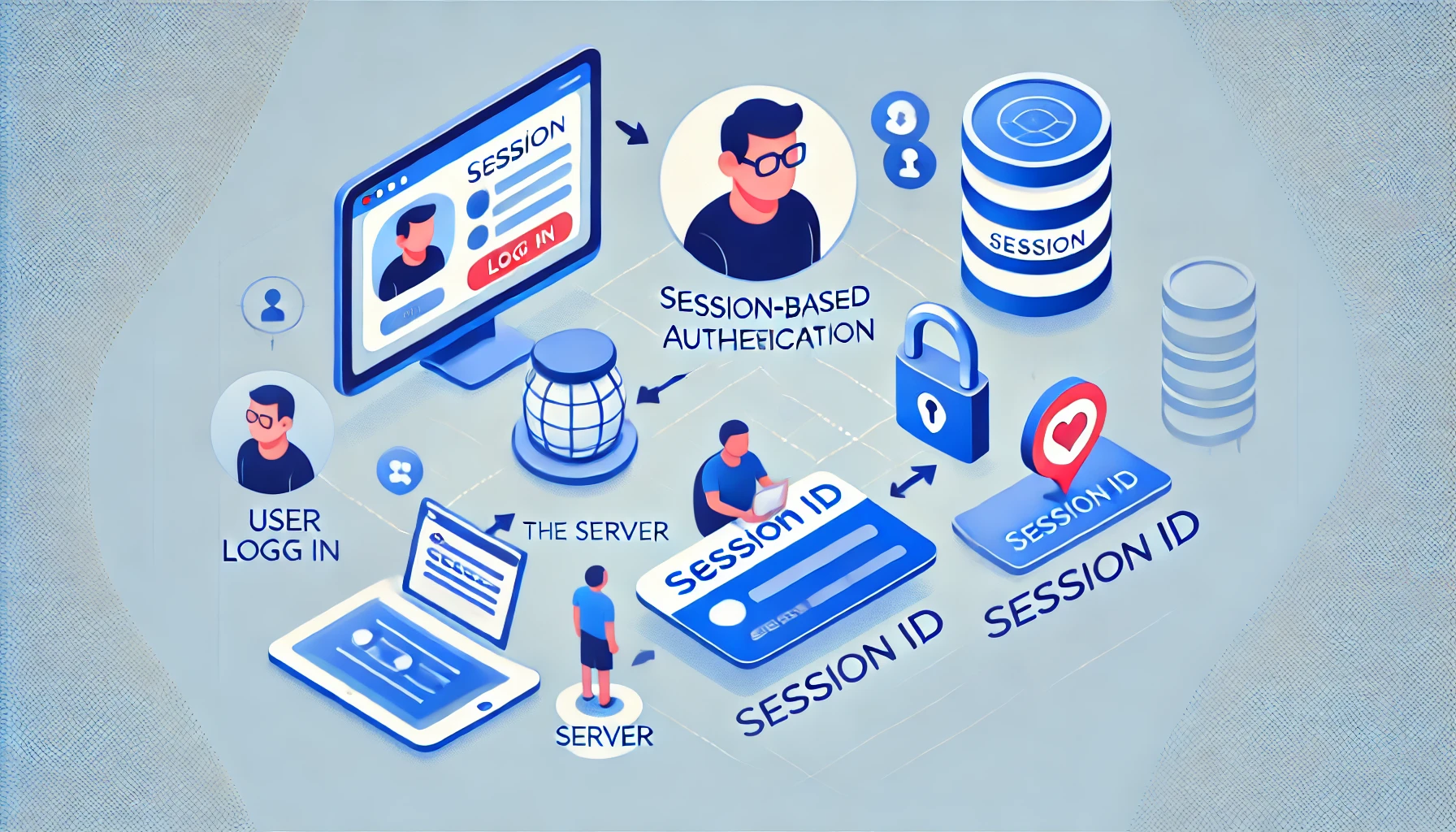
How It Works:
- A user logs into your web app.
- The backend server verifies the login details and creates a session.
- The server stores the session information in a session store (a database or memory).
- The server sends back a session ID as a cookie.
- For every subsequent request, the session ID is sent along with the request.
- The server checks the session store for the session ID to verify the user.
Here’s an example in code:
app.post('/login', (req, res) => {
const sessionId = createSession(req.body.userId); // Create session
res.cookie('sessionId', sessionId); // Send session ID in cookie
res.send('Login successful');
});
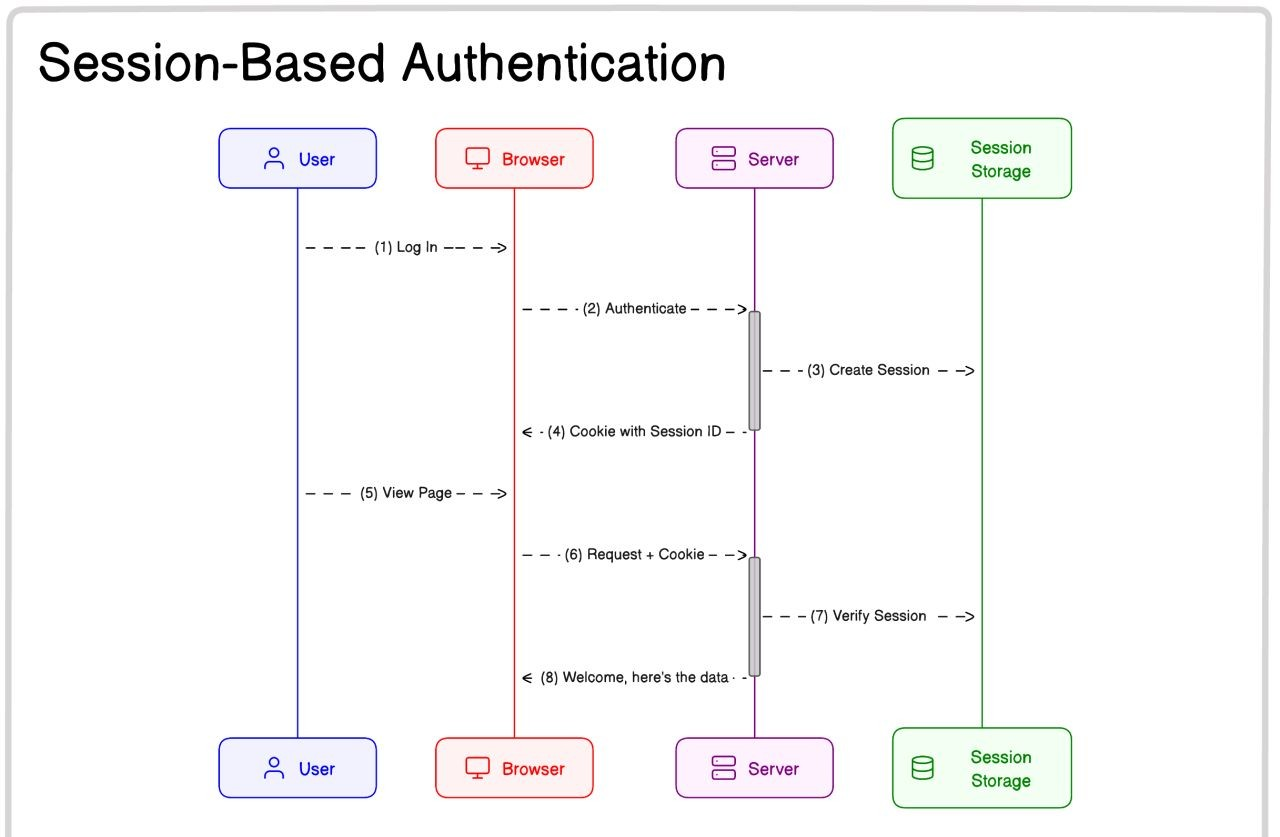
JWT-Based Authentication
JWT (JSON Web Token)-Based Authentication does not store any session data on the server. Instead, all the required user information is included within the JWT itself, like carrying your entire flight ticket with all your details encrypted in a safe format.
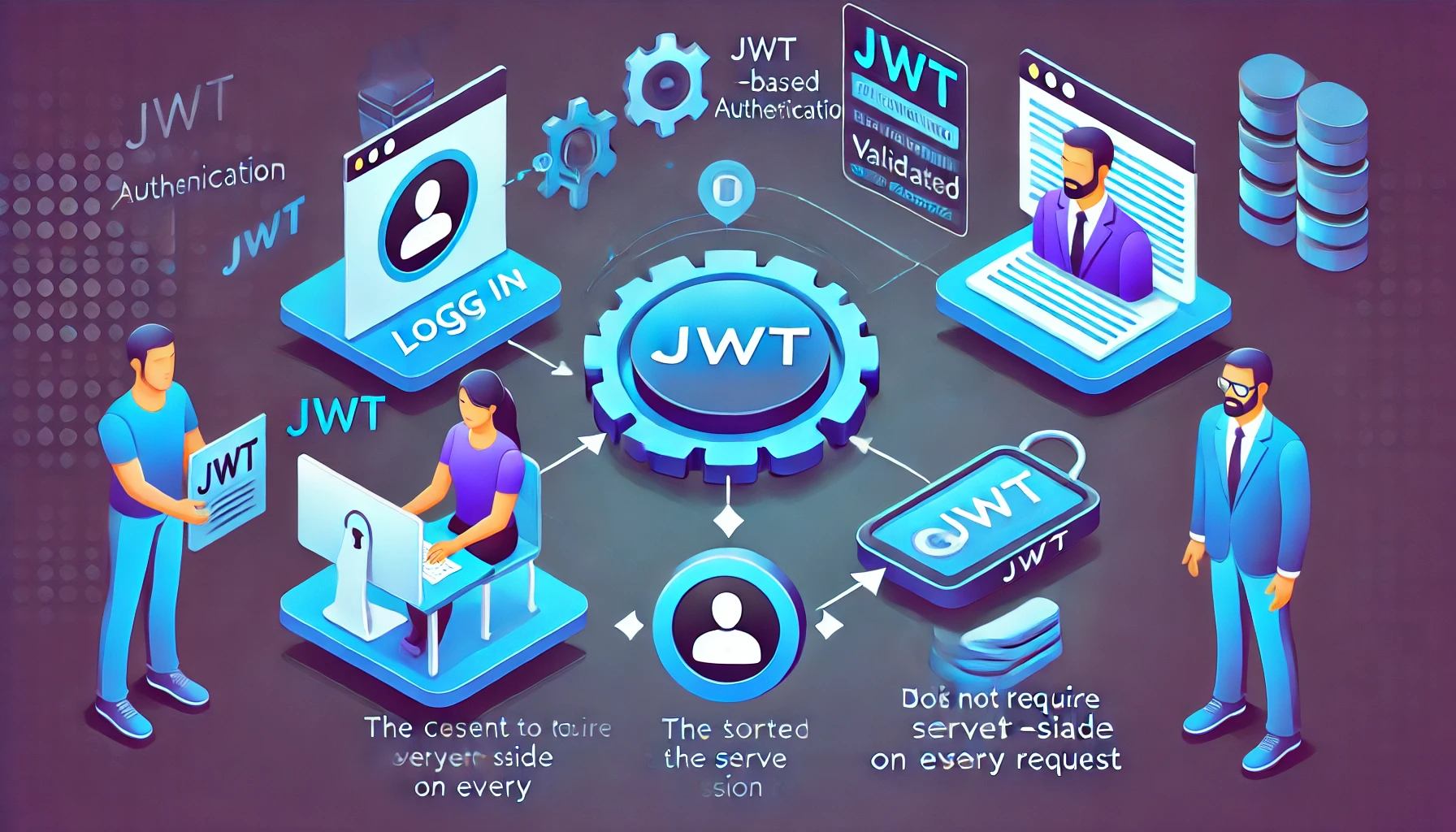
How It Works:
- A user logs into your web app.
- The backend server verifies the credentials and creates a JWT, signing it with a private key.
- The JWT is sent to the client as a cookie.
- For every subsequent request, the JWT is sent along with the request.
- The server validates the JWT by verifying its signature and extracts user info from the token itself.
Here’s how it looks in code:
app.post('/login', (req, res) => {
const token = createJWT(req.body.userId); // Create JWT
res.cookie('token', token); // Send JWT in cookie
res.send('Login successful');
});
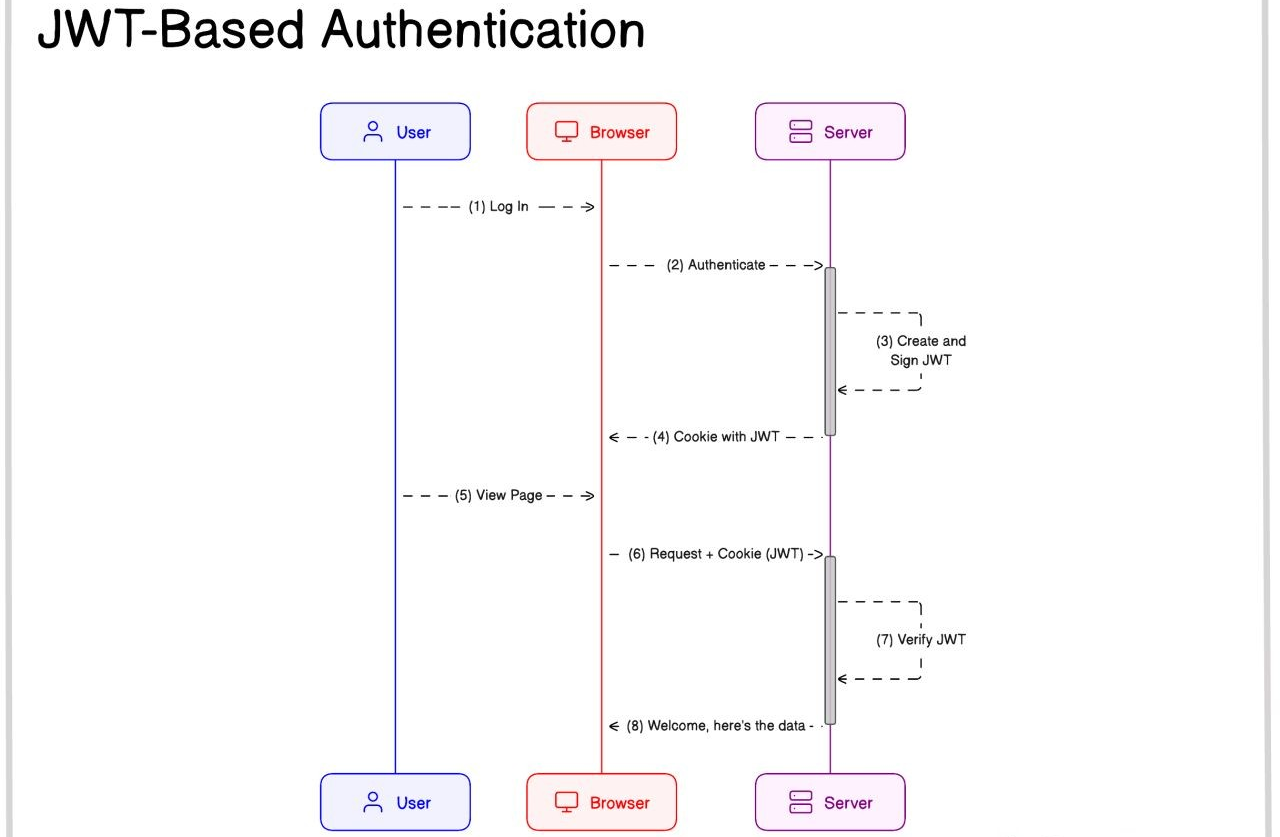
Session-Based Authentication vs JWTs
Session-Based Authentication Pros:
- Server-Side Control: You can easily invalidate a session by removing it from the session store.
- Smaller Payload: Since only a session ID is sent with each request, it consumes less bandwidth.
Session-Based Authentication Cons:
- Scaling Issues: Session stores need to be synchronized across multiple servers, which can introduce complexity.
JWT-Based Authentication Pros:
- Stateless: Since all data is stored in the token itself, there’s no need for server-side session storage, making it easier to scale.
- Decentralized: JWTs work well in distributed systems where different services might need to verify the user independently.
JWT-Based Authentication Cons:
- Difficult to Invalidate: Once issued, JWTs cannot be easily revoked. You may need to implement a blacklist or token expiration to counter this.
- Larger Payload: JWTs can grow in size, which could impact performance, especially with large payloads.
Which One Should You Use?
The answer, like many things in software development, is: It depends.
- If your app is relatively small, needs centralized control, or you want easy session invalidation, Session-Based Authentication might be the better choice.
- If you need a stateless, scalable solution for a microservices architecture, JWTs can offer more flexibility.
Conclusion
Both Session-Based Authentication and JWT-Based Authentication have their strengths and weaknesses. Understanding your app’s requirements will help you choose the right authentication strategy. If you’re building a large, distributed system, JWT might be the better fit. If you prioritize simplicity and easy session management, go with Session-Based Authentication.
Whether you choose sessions or JWTs, always prioritize security. Keep tokens safe, use HTTPS, and make sure you implement mechanisms like token expiration or session timeouts to protect your users.
Feel free to dive deeper into either approach by experimenting with code examples, and decide based on your app’s specific needs. If you’re building a new app, choosing the right authentication mechanism from the start can save you a lot of headaches down the road.